The Brane SDK represents a fundamental shift in application development for high-performance computing. Unlike conventional development kits that force developers to maintain separate codebases for different hardware targets, the Brane SDK embraces the heterogeneous computing paradigm—allowing you to write code once and deploy it efficiently across a diverse range of computing architectures.
This unique capability means your applications can seamlessly leverage the specific strengths of different hardware platforms: the general-purpose processing power of CPUs, the massive parallelism of GPUs, the deterministic execution of Kalray MPPAs, the customizable logic of FPGAs, and the scalable resources of cloud environments. By supporting this cross-platform flexibility, the Brane SDK helps you maximize performance while minimizing development effort.
Supported Programming Models and Languages #
The Brane SDK achieves its remarkable flexibility through comprehensive support for multiple programming models, each designed to excel in specific computing contexts:
Cross-Platform Parallel Computing #
OpenCL provides a standardized framework for writing programs that execute across heterogeneous platforms. It offers a consistent programming interface that works across CPUs, GPUs, and accelerators from different vendors, enabling truly portable parallel code.
GPU-Specific Acceleration #
AMD HIP (Heterogeneous-Computing Interface for Portability) allows developers to write GPU code that can run on both AMD and NVIDIA hardware with minimal changes, providing excellent performance portability.
NVIDIA CUDA offers deep integration with NVIDIA’s GPU architecture, enabling maximum performance for applications running on NVIDIA hardware.
CPU Parallelization #
POSIX Threads provides low-level threading capabilities with fine-grained control over thread creation, synchronization, and management—ideal for applications requiring precise control over execution.
OpenMP simplifies parallel programming through compiler directives, making it easy to parallelize existing code with minimal changes while maintaining readability.
FPGA Development #
Hardware Description Languages (HDLs) enable direct hardware design for FPGAs:
Verilog offers a C-like syntax for hardware description and is widely used for digital design.
SystemVerilog extends Verilog with advanced verification features and object-oriented programming constructs.
VHDL provides a strongly-typed approach to hardware description with robust type checking and formalized methodology.
This diverse language support ensures you can choose the most appropriate programming model for each component of your application, optimizing both development efficiency and runtime performance.
The Brane SDK Development Environment #
While the Brane SDK’s core functionality works with any development environment, it provides particularly deep integration with the IntelliJ IDE. This integration creates a seamless development experience with specialized tools for heterogeneous computing. The following sections will guide you through this environment, though the fundamental concepts apply equally to other IDEs or command-line development.
The Brane-Builder System: Streamlining Multi-Platform Development #
At the heart of the Brane SDK lies the Brane-Builder—a sophisticated build system that extends Gradle to support heterogeneous computing. This architecture transforms the traditionally complex process of multi-platform development into a streamlined workflow with several key advantages:
Unified Development Environment
The Brane-Builder eliminates the need to maintain separate codebases for different target platforms. You can develop your application once and deploy it across all supported architectures, from CPUs and GPUs to FPGAs and cloud environments. This unified approach dramatically reduces code duplication and maintenance overhead while ensuring consistent behavior across platforms.
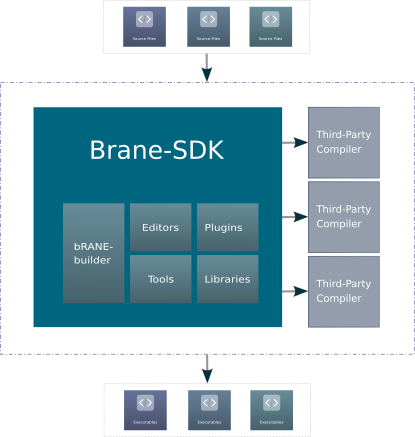
Rapid Iteration Cycle
Development velocity benefits enormously from the instant compilation capabilities of the Brane-Builder. Changes to your code can be tested immediately, shortening the feedback loop and enabling rapid iteration. This quick turnaround time allows for more experimentation and refinement, ultimately leading to better application design and performance.
Modular Application Architecture
Complex applications often require multiple components working together. The Brane-Builder supports this through its multi-project capabilities, allowing teams to work on separate modules simultaneously and integrate them seamlessly. This modular approach improves code organization, enables parallel development, and facilitates code reuse across projects.
Integrated Development Toolchain
The Brane SDK integrates debugging and performance profiling directly into the development environment. These tools help identify and resolve issues efficiently, with specialized support for the unique challenges of heterogeneous computing. The integrated approach means you don’t need to switch between different tools for development, debugging, and optimization.
Collaborative Development Support
Modern software development is inherently collaborative. The Brane SDK provides seamless Git integration for version control, branch management, and team collaboration. This integration streamlines the collaborative workflow and ensures that all team members can work effectively together on complex heterogeneous computing projects.
Performance Visibility
Understanding application performance across different architectures can be challenging. The Brane-Builder generates detailed compilation reports that highlight performance bottlenecks and compatibility issues across different target platforms. These insights help you optimize your code for each specific architecture while maintaining cross-platform compatibility.
Project Organization and Structure #
The Brane SDK adopts familiar project organization principles to minimize the learning curve for developers. This structure follows conventions used in major IDEs like IntelliJ and Eclipse, making it accessible to developers with experience in those environments.
Module Types #
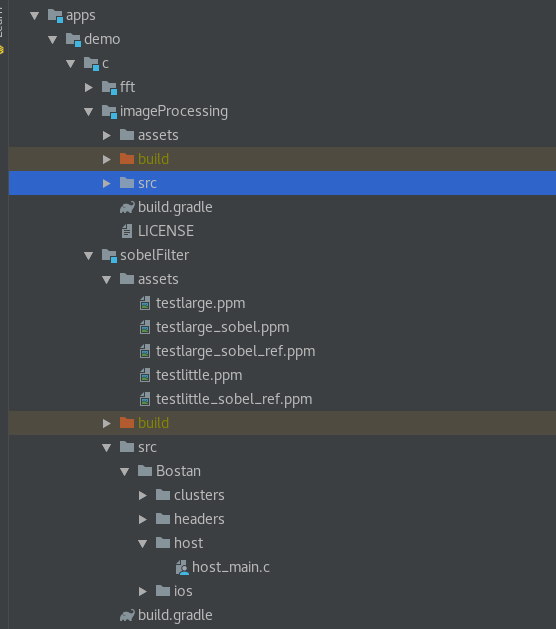
Each project in the Brane SDK contains one or more modules, which serve as the building blocks of your application. The SDK supports two primary types of modules:
- Application Modules are self-contained executable programs that perform specific functions. For example, a “sobelFilter” module might implement an image processing algorithm that applies the Sobel operator for edge detection.
- Library Modules contain reusable code collections that can be shared across multiple application modules. These modules promote code reuse and modular design, allowing you to build complex applications from well-defined, testable components.
The SDK organizes these modules in the Project View, providing a hierarchical representation that makes it easy to navigate through your source files and resources.
Important: Maintaining the project hierarchy structure as presented in the SDK is crucial for avoiding bugs and compilation issues. The build system relies on this structure to correctly identify and process your source files.
Standard Directory Structure #
Each module follows a standardized directory structure that helps organize your code and resources:
- build.gradle: The configuration file at the top level of each module that defines build settings, dependencies, and target platforms.
- assets/: A directory containing non-code resources required by your application, such as images, data files, and configuration settings.
- src/: The directory containing all source code files, organized by language (C, C++, HDL) and purpose.
- build/: The directory where compiled executables and intermediate files are stored during the build process.
Note: The project structure on your disk may differ from the flattened representation shown in the Brane SDK interface. The IDE organizes the view for development convenience, abstracting away some of the underlying file system complexity.
You can customize your project view to focus on specific aspects of development. For example, switching to the “Problems” view displays links to source files containing recognized coding and syntax errors, helping you quickly identify and fix issues in your codebase.
Navigating the Brane SDK Interface #
The Brane SDK leverages the IntelliJ IDE to provide a comprehensive development environment. Understanding the key interface elements will help you navigate and use the SDK efficiently.
Main Window #
The main window consists of seven important logical areas, each serving a specific purpose in the development workflow:
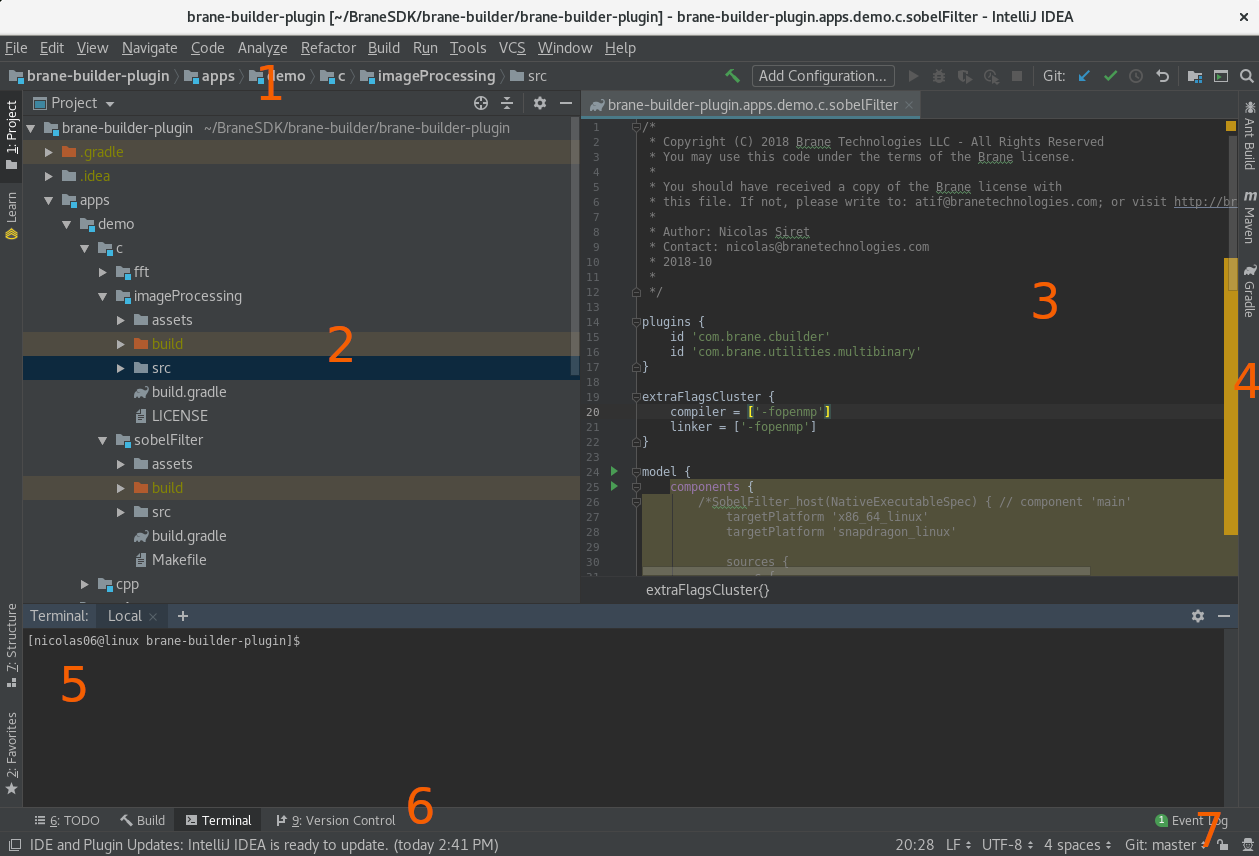
Over all the logic areas, the seven presented in the figure 4 are the most important ones:
- Toolbar: Provides quick access to common actions including refactoring, building modules, and launching tools. The toolbar can be customized to include your most frequently used commands.
- Project View: Serves as a navigation hub for your project, allowing you to browse through modules, directories, and files. Different view modes (Project, Problems, Structure) offer alternative perspectives on your codebase.
- Editor Window: The central workspace where you create and modify code. The editor adapts based on file type, providing language-specific features such as syntax highlighting, code completion, and error detection.
- Tool Window Bar: Runs around the outside of the IDE window, containing buttons that expand or collapse individual tool windows. This allows you to maximize screen space while keeping essential tools accessible.
- Terminal: Provides command-line access for executing specific tasks such as compiling modules, creating execution files, and running custom commands that may not be available through the GUI.
- Tool Windows: Offer specialized interfaces for various development tasks and information displays, including debugging, version control, build output, and code analysis results.
- Event Log: Maintains a chronological record of all significant events that have occurred during your SDK session, helping you track actions and diagnose issues.
You can customize the main window layout to optimize your screen space by hiding or repositioning toolbars and tool windows. Most IDE features can also be accessed through keyboard shortcuts for efficient workflow, reducing the need for mouse interactions.
Key Features and Shortcuts #
The Brane SDK inherits IntelliJ’s powerful code navigation and editing capabilities. Mastering these features can significantly enhance your development productivity, especially when working with complex heterogeneous computing projects.
Search and Navigation
One particularly useful feature is the ability to search across your entire project by double-pressing the Shift key. This action activates the “Search Everywhere” function, allowing you to quickly find any file, class, symbol, or action within your project. This universal search capability is invaluable when working with large projects spanning multiple modules and target platforms.
Code Completion Types
The SDK offers three sophisticated types of code completion that accelerate development while reducing errors:
Type | Description | Keyboard Shortcut |
Basic Completion | Displays suggestions for variables, types, methods, and expressions. Pressing the shortcut twice shows more results, including private members and non-imported static members. | Control+Space |
Smart Completion | Provides context-aware suggestions based on expected types and data flows. Pressing the shortcut twice shows more results, including chains of method calls. | Control+Shift+Space |
Statement Completion | Automatically completes code constructs by adding missing parentheses, brackets, braces, and proper formatting. | Control+Shift+Enter |
These completion mechanisms are particularly valuable when working with complex APIs and multiple programming models, as they help ensure correct usage and reduce syntax errors.
For quick fixes and intention actions (smart suggestions to improve your code), simply press Alt+Enter when the cursor is positioned on code that has available actions. This shortcut provides context-aware recommendations that can help refactor code, optimize performance, and resolve potential issues.
Essential Navigation Commands #
Efficient navigation is critical for productive development, especially in heterogeneous computing projects that often involve multiple source files across different modules. Here are the most frequently used navigation commands:
Action | Description | Keyboard Shortcut |
View File Structure | Display the structure of the current file, showing classes, methods, and fields | Control+F12 |
Use Bookmarks | Create an anonymous bookmark at the current line | F11 |
Open Bookmarks | View and navigate to all bookmarks | Shift+F11 |
Recent Files | Switch between recently accessed files | Control+E |
Navigate to Class | Search for and navigate to a specific class | Control+N |
Navigate to File | Navigate to any file or folder in the project | Control+Shift+N |
These navigation features help you move efficiently through your codebase, reducing the time spent searching for specific components and allowing you to focus on development tasks.
For a more visual introduction to navigation features, you can watch this helpful tutorial: IntelliJ Website.
Reformat and rearrange code #
Consistent code formatting improves readability and reduces maintenance challenges, especially in collaborative projects. As you edit, the Brane SDK automatically applies formatting and styles as specified in your code style settings.
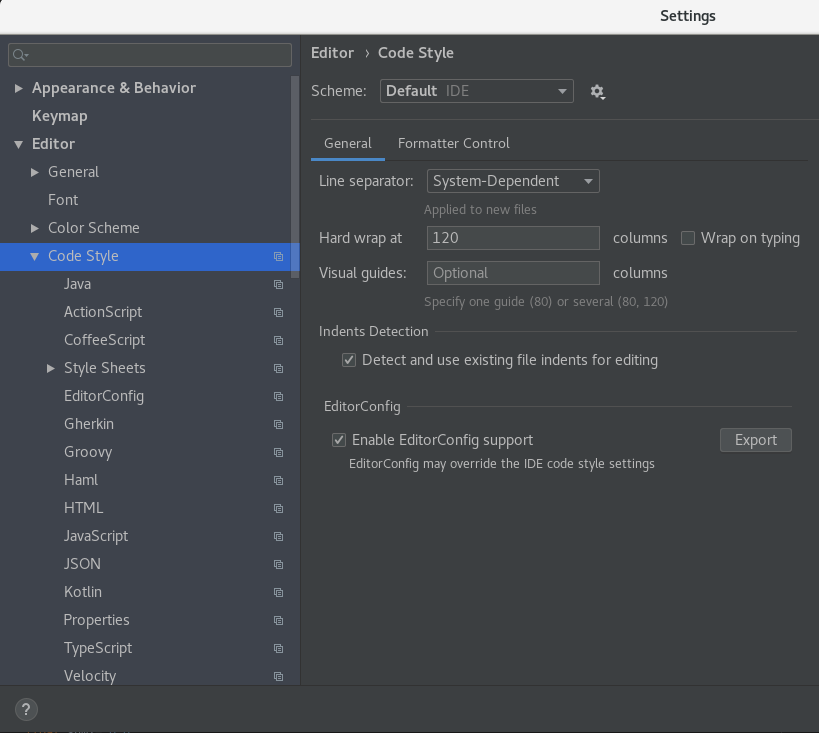
To customize your code style settings, Press Ctrl+Alt+S
and go to Editor
and Code Style
.
You can customize the code style settings by programming language, including specifying conventions for tabs and indents, spaces, wrapping and braces, and blank lines.
You can reformat a part of code, the whole file, group of files, a directory, and a module. You can also exclude part of code or some files from the reformatting.
Although the IDE automatically applies formatting as you work, you can also explicitly call the Reformat Code action by pressing Control+Alt+L
,or just reformat a code fragment in a file by select a code fragment and press Ctrl+Alt+L
. You can also auto-indent all lines by pressing Control+Alt+I
. You will find more information tips, and tricks on the IntelliJ Website.
The Brane-Builder Architecture #
The Brane-Builder forms the foundation of the Brane SDK, providing a sophisticated build system specifically designed for heterogeneous computing applications. It extends the standard Gradle build system with Brane-specific plugins and optimizations that streamline the development process.
Key Capabilities #
The Brane-Builder enables developers to:
- Compile efficiently: Build application and library modules with optimizations for both development speed and production performance.
- Configure for specific targets: Extend build processes to target specific hardware platforms with customized compilation flags, optimization levels, and platform-specific code.
- Generate deployable assets: Create executables, installers, and multi-binary files tailored for diverse architectures, ensuring compatibility across deployment environments.
- Manage dependencies: Leverage Gradle’s powerful dependency management to incorporate external libraries and internal modules seamlessly.
Build Configuration #
The build system uses Gradle’s command-line tools and relies on a build.gradle
configuration file to define the project structure and build processes. This script contains several key elements:
- Project structure definitions that specify the organization of your code
- Task configurations that define the build steps for different targets
- Dependency declarations that identify required libraries and modules
- Build options specific to target platforms that customize the compilation process
While detailed instructions on writing build scripts will be covered in dedicated sections later in this documentation, the basic structure follows standard Gradle conventions with Brane-specific extensions. This approach allows developers familiar with Gradle to quickly adapt to the Brane-Builder system while providing powerful capabilities for heterogeneous computing.
Dependency Management #
Dependencies in your project are specified in the build.gradle
file and are automatically resolved and managed by Gradle. The system supports several types of dependencies:
- Module Dependencies: These are references to other modules within the same project, allowing you to create modular applications with well-defined interfaces between components.
- Remote Binary Dependencies: Libraries downloaded from repositories like Maven Central or JCenter, providing access to a vast ecosystem of existing code.
- Local Binary Dependencies: Custom libraries stored in your project or on your machine, useful for integrating proprietary or specialized components.
The Brane-Builder handles all aspects of dependency management, including downloading, versioning, conflict resolution, and integration. This comprehensive approach simplifies the development process and ensures consistent behavior across different environments.
Debugging and Performance Analysis #
The Brane SDK includes tools to help you debug your code and optimize its performance across different hardware targets. These tools provide insights into application behavior, helping you identify and resolve issues efficiently.
Many advanced debugging and profiling features are still under active development at Brane, reflecting the evolving nature of heterogeneous computing tools. While these cutting-edge capabilities are not included in the standard SDK release, they are available through an early access program. To gain access to these tools, you can send an email request to Brane’s support team.
Compilation information #
When working on a module, you can obtain detailed insights into the compilation process using Gradle’s build scan feature. The Brane SDK leverages this capability to provide comprehensive compilation analytics that help you understand and optimize your build process.
To activate this feature, simply add the --scan
option when running your build command:
$ gradle assemble --scan
This command will compile all your modules and generate a build scan report. After compilation completes, you’ll receive a link to access detailed information including:
- Timeline of the build process, showing the sequence and duration of tasks
- Task execution details, including inputs, outputs, and dependencies
- Project structure information, mapping the relationships between components
- Performance metrics that identify bottlenecks in the build process
- Dependency insights showing how libraries are resolved and integrated
- And many other valuable metrics and visualizations
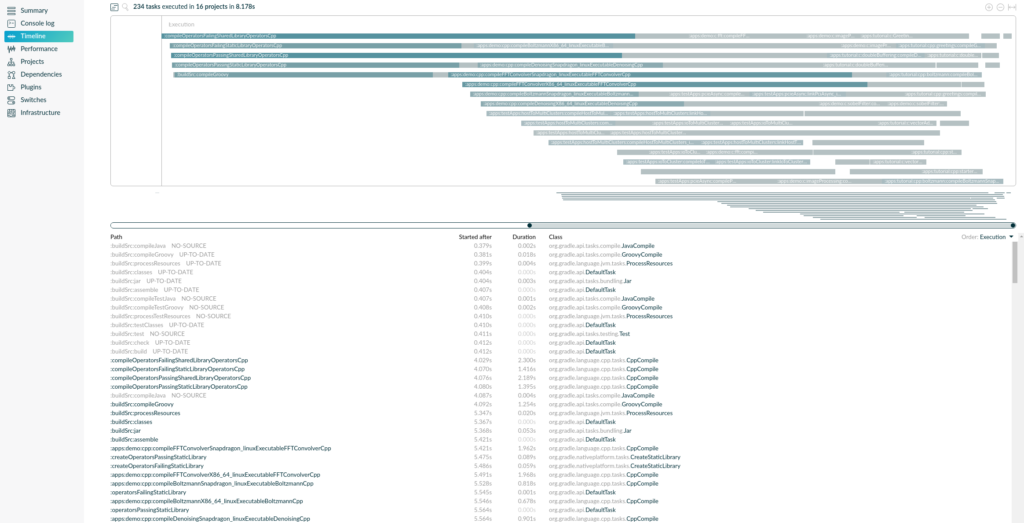
This information can be invaluable for diagnosing build issues, optimizing compilation times, and understanding the relationships between components in your project. By analyzing these insights, you can make informed decisions about project structure, dependency management, and build configuration.
Next Steps #
Now that you understand the fundamentals of the Brane SDK and its development environment, you can begin exploring its more advanced capabilities. Here are some suggested next steps to deepen your knowledge and expand your development skills:
- Explore advanced configurations for multi-platform development in the following chapters, where we’ll cover platform-specific optimizations and cross-compilation strategies.
- Customize your application logic by modifying template files like
main.c
to implement your specific computational requirements and algorithms. - Build modular applications by integrating additional modules, libraries, and dependencies, creating scalable solutions that leverage the full power of heterogeneous computing.
- Master performance optimization techniques that we’ll cover in upcoming sections, including hardware-specific tuning, parallelization strategies, and memory management best practices.
- Apply debugging methodologies tailored for multi-platform applications, helping you identify and resolve issues that may appear differently across various hardware targets.
- Study real-world case studies and application examples that demonstrate how to solve common heterogeneous computing challenges using the Brane SDK.
The following sections will dive deeper into these topics, providing practical examples, detailed tutorials, and comprehensive reference materials to support your development journey with the Brane SDK.